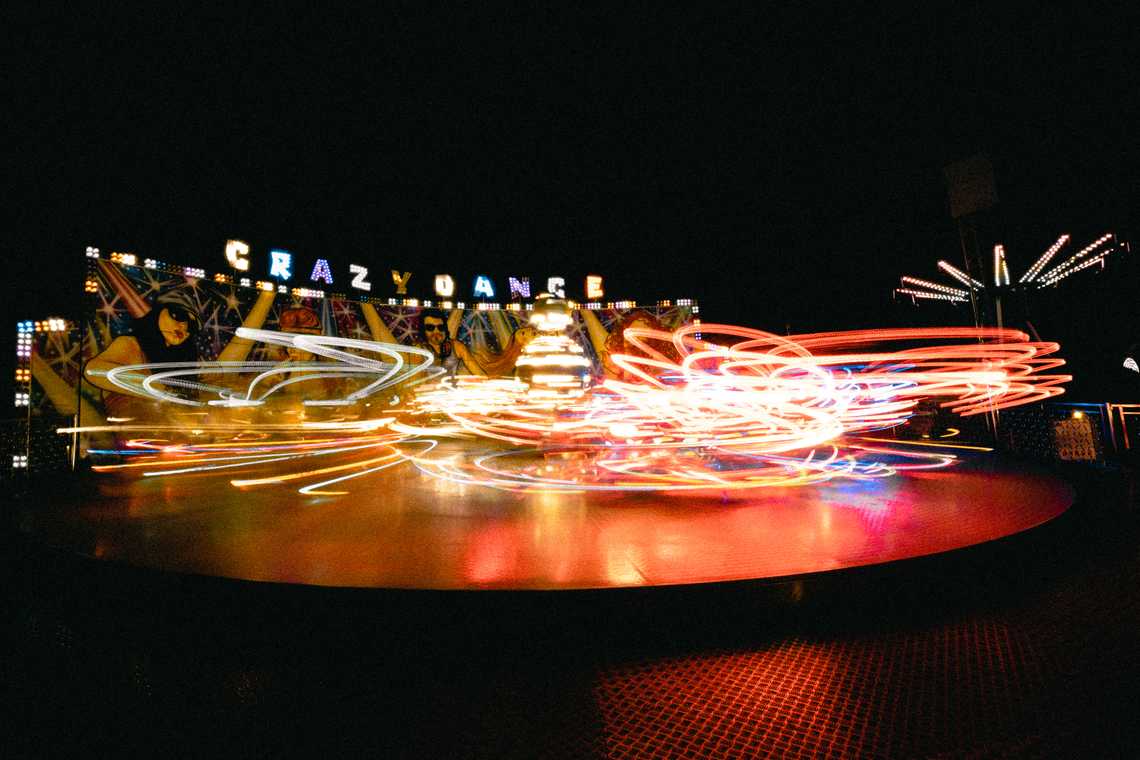
View Transitions in Oracle APEX
Web Animations
#From Documents to Web Apps
#The web is perhaps one of the greatest examples of improving something instead of creating something new. It started with plain white documents, black text, and a few blue anchors. Anchors may still be blue, but the web is now a ubiquitous platform for almost anything.
I remember visiting my favorite games’ website ~15 years ago and waiting almost a minute for anything to load while the modem was making acoustical R2D2 impressions. People around me also did not use the German term for “loading”, instead referring to “building up” the page. This was because you could follow the rendering from top to bottom.
Nowadays, good websites load instantly.
Animations make UIs polished
#While computing power increased, developers started to add motion to the UIs of native programs. This does not only look exciting and engaging but can also give the user important context. Like when you enter the wrong pin into your phone and the UI shakes. Or transitions can give us the feeling that the UI is something physical where we move around.
The material design motion guidelines are a great resource to learn more about animations and their benefits. And they have a lot of pretty good examples:
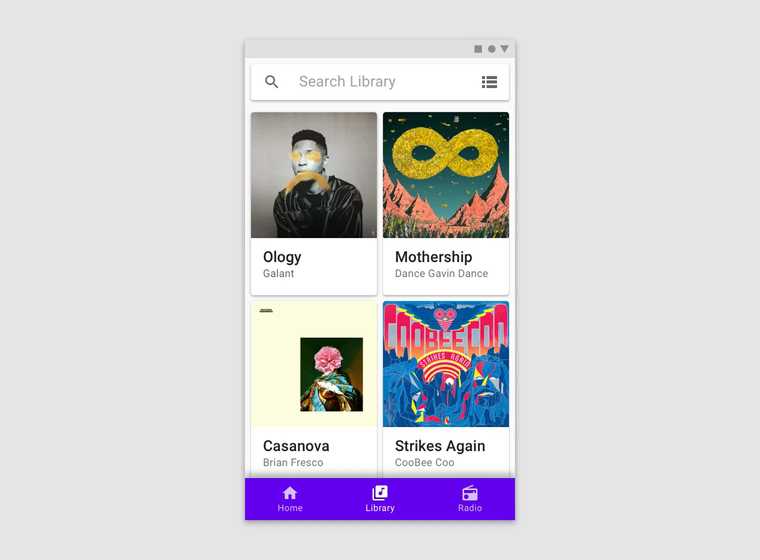
The web lacks behind
#As any phone app has nice animations and transitions nowadays, I think the web may fall behind. For simple text sites, it is not a big deal, but polished web apps like YouTube, the Spotify web app, or the Apple Store use animations rarely.
I think many developers are not really aware of what is possible. Additionally, complex animations are quite hard to get to work. The examples on MDN even cause a horizontal scrollbar to appear.
In addition to plenty of CSS properties, there are even full libraries. I remember checking out react-spring but looking at the logic required to do stuff, I quickly lost interest. Maybe it needs some opinionated default styles that just work like on-visible: slide-in right 0.5s;
.
And another thing was not even possible until recently: transitioning from one page / view to another. This is what I want to show you today.
View Transitions API
#This API was introduced by Google in August 2021. Jake Archibald wrote a great blog post where he shows the capabilities and how to use them. Here is a video of the example he created:
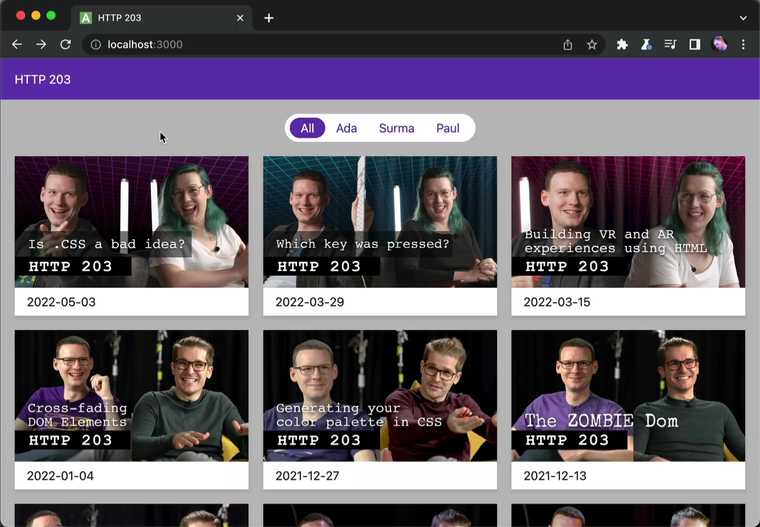
The API was submitted as a draft to the W3C to become a Web standard. It landed in the Chromium browser with version 111 but is currently (as of September 2023) missing in WebKit and Firefox. The good news is that we can use it today, as unsupported browsers just ignore the statements. Let’s hope that other browsers will adapt this soon.
The only problem is that this API only works for single-page applications. This means only apps that use JavaScript to change the page content dynamically can use this feature. APEX, on the other hand, loads a new document when you click on a link (multipage application / server-side rendering). But luckily, Google is currently experimenting with server-side-rendered page transitions.
Since May 2023, they have been working on a spec that describes the API for cross-document transitions. And with experimental flags for Chrome, we can try this out for APEX apps.
View Transitions in APEX
#Default page transitions
#To use the transitions, we need to add a meta tag to the header of the HTML. We can add this to the HTML header attribute of a page.
1<meta name="view-transition" content="same-origin" />
2
The spec states that in the long term, a CSS property will be better suited. This allows for media queries to control behavior and, in my opinion, is more handy as styles belong to CSS.
1@auto-view-transitions {
2 same-origin: enabled;
3}
4
The meta tag is also difficult to add to every page. One option would be to modify / create page templates. But I do not recommend doing this because they may be changed by Oracle in the future, and then you are on your own to make sure that everything still works.
After adding the tags, we already get this nice transition when we navigate between pages with the meta tag:
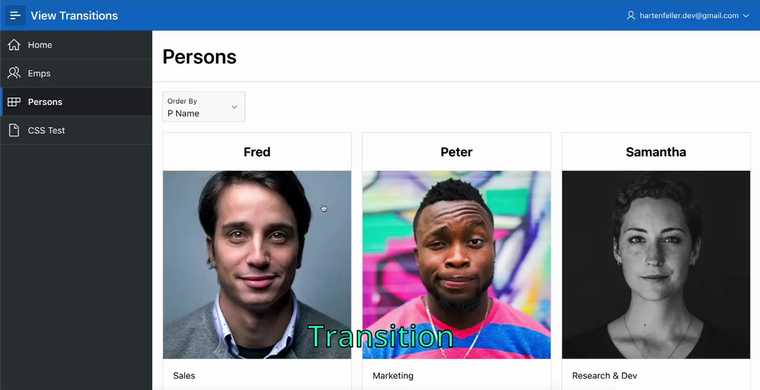
Modify the transition
#We can modify how the animation looks by defining CSS key frames. As I have no clue how to make great animations, I just copied one from the Google blog post that implements a transition from the material design guidelines. It lets the old page fade out to the left and the new one fade in from the right.
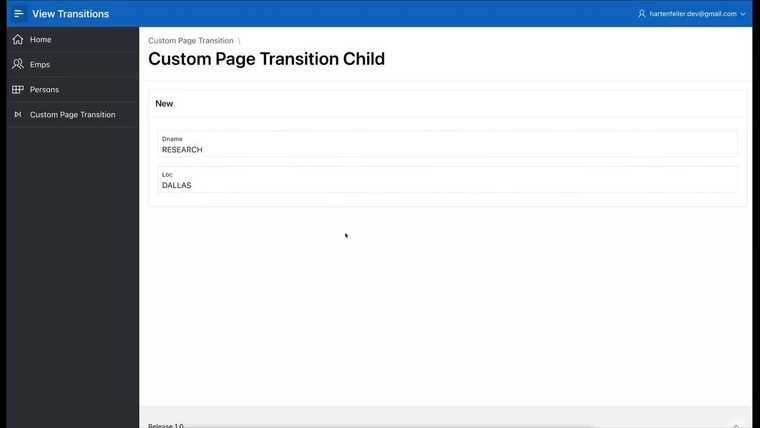
(The white stripes in the video on the left are compression artifacts.)
The CSS is the following:
1@keyframes fade-in {
2 from {
3 opacity: 0;
4 }
5}
6
7@keyframes fade-out {
8 to {
9 opacity: 0;
10 }
11}
12
13@keyframes slide-from-right {
14 from {
15 transform: translateX(30px);
16 }
17}
18
19@keyframes slide-to-left {
20 to {
21 transform: translateX(-30px);
22 }
23}
24
25::view-transition-old(root) {
26 animation: 90ms cubic-bezier(0.4, 0, 1, 1) both fade-out, 300ms cubic-bezier(
27 0.4,
28 0,
29 0.2,
30 1
31 ) both slide-to-left;
32}
33
34::view-transition-new(root) {
35 animation: 210ms cubic-bezier(0, 0, 0.2, 1) 90ms both fade-in, 300ms
36 cubic-bezier(0.4, 0, 0.2, 1) both slide-from-right;
37}
38
Browser magic: transitioning specific elements
#We can assign a CSS property to specific elements on both the source and destination and let the browser automatically create a transition from the first position to the second. The browser just compares the positions of the elements with the same transition name on both pages and animates the position change.
Elements with this setting overwrite the default page transition. When we add this to the static header and navigation bar, which stay at the same position, only the body content is animated:
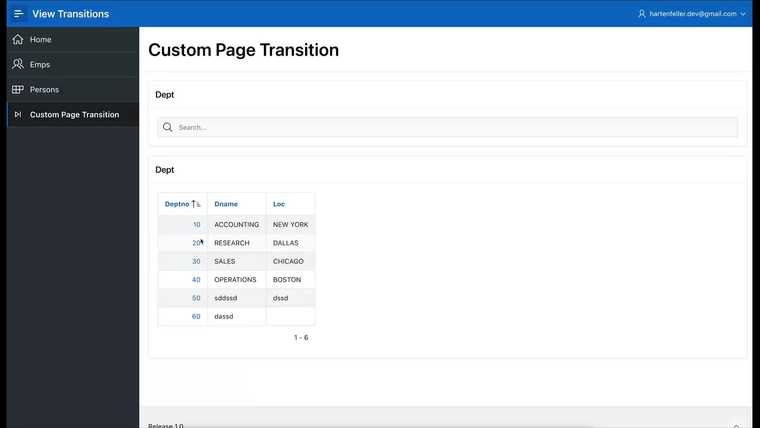
1.t-Body-nav {
2 view-transition-name: navigation-menu;
3}
4
5.t-Header {
6 view-transition-name: app-header;
7}
8
We can also use this to animate text or media that is present on both pages:
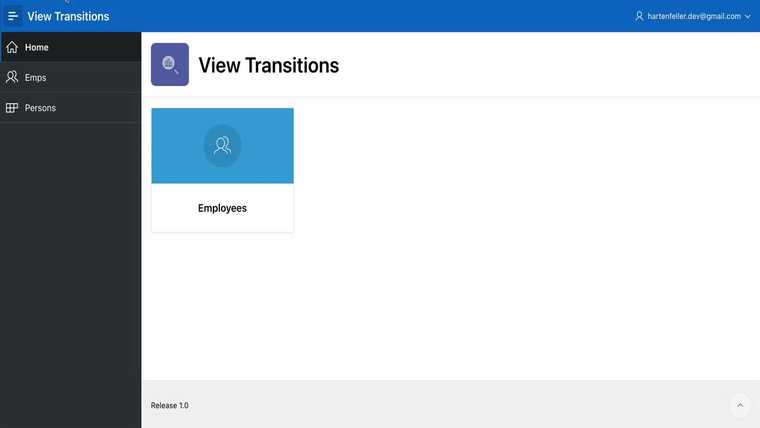
The first example is basic. We just need to add view-transition-name
properties to the card title and the page breadcrumb:
1/* card page */
2#p1-cards .t-Cards-item:first-child .t-Card-title {
3 view-transition-name: emp-header;
4}
5
6/* emp page */
7#p2-header .t-Breadcrumb-label {
8 view-transition-name: emp-header;
9}
10
In the second example, we transition from any clicked employee to the detail page. In this case, we can’t just add the same transition name to each employee’s name. The browser does not compare the text and then determine we want to transition “Blake” to “Blake”. Instead, it just notices that there are multiple elements with the same transition name and does nothing.
But of course, we can fix this by using unique transition names. In the “ENAME” column, I used the following HTML expression:
1<span style="view-transition-name: emp-name-#EMPNO#">#ENAME#</span>
2
On the target page, we can just reference the page item with the ID in the page inline styles:
1#R15375539247587326948_heading {
2 view-transition-name: emp-name-&P3_EMPNO.;
3}
4</style>
5
The third example with the images looks the best, in my opinion. It works the same way. It is quite tricky to get the transition name property into the card image. I copied the HTML structure and enabled “Advanced Formatting”. This is quite a bad solution, as it could break in the future.
1<div class="a-CardView-media a-CardView-media--body a-CardView-media--cover">
2 <img
3 class="a-CardView-mediaImg"
4 src="&P_IMAGE_URL."
5 alt=""
6 loading="lazy"
7 style="view-transition-name: image-&P_ID.;"
8 />
9</div>
10
Bleeding Edge
#This is at an early stage, and the API can and probably will change. It can take years before this is a standard, and even longer until it is supported by all browsers. I really hope that the transitions for server-side pages (we need for APEX) will be accepted in the same W3C specification and land in other browsers at the same time. The transition API for client-side rendered pages is pretty stable and already used in the wild and supported by web frameworks.
For the server-side API, there are currently problems with the element transitions. They do not work consistently (at least on my machine). I had to heavily cut the video to show only the working examples. Also, the text transitions look a little bit quirky.
You also need to enable an experimental flag in Chrome to get this to work. Paste this into the Chrome URL bar, activate the flag, and restart the browser:
1chrome://flags#view-transition-on-navigation
2
Then you can check out my demo app for yourself.
This should be an APEX feature in the future
#When the spec is stable and maybe multiple browsers fully support it, I guess this should be a feature in APEX with a low-code interface. I guess many web apps will use view transitions in the next few months and years, and APEX should not fall behind. But APEX is also famous for stability and reliability, so I think they should wait until the specification is out of the early phases.