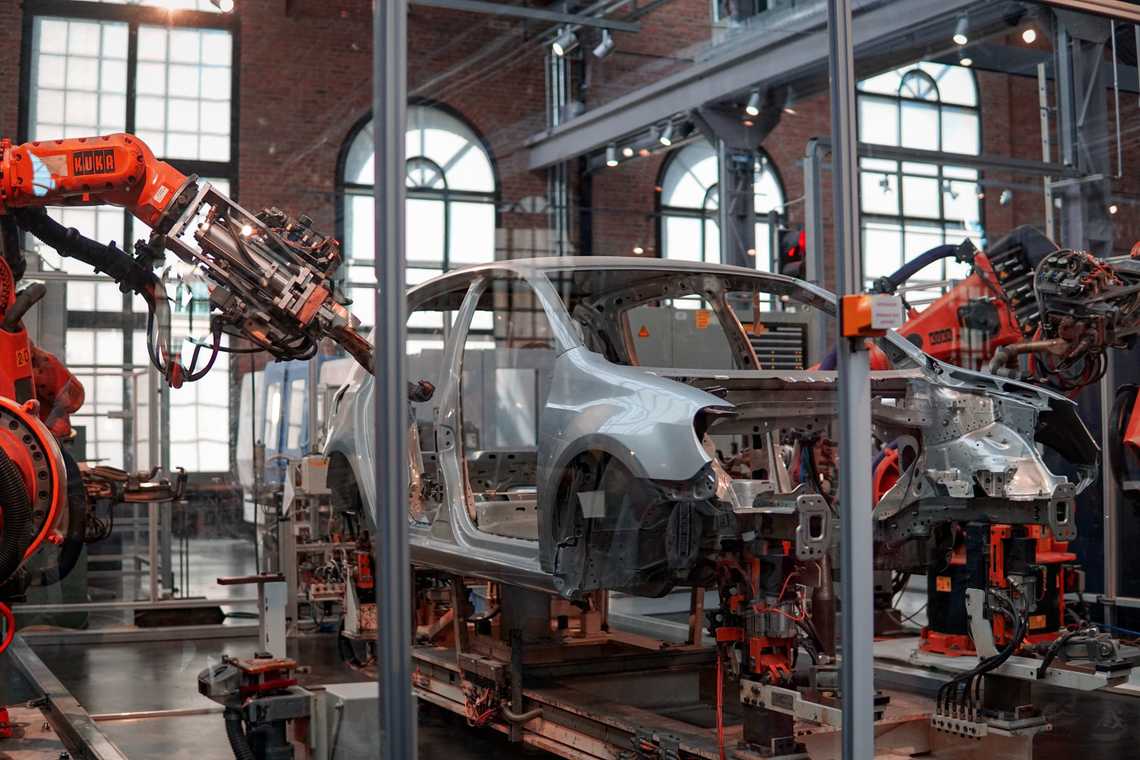
GitHub Actions Plugin for Cronicle
Environment specific tasks
#For many automation like Unit Tests, you can run your code exclusively in the environment of GitHub with their actions. For things specific to your environment, you might need something to run on your server. For example, if I push to my website’s GitHub repo I want to build the website and deploy it as a private preview on my server.
For requirements like this GitHub offers self-hosted runners but for me, they are too complex to set up, limited to one repository (at least back when I used them), and too much of a black box for my taste.
For automated tasks on my server, I use Cronicle which is a small job server with a small web UI. It’s pretty simple to use, just create a Job and add a shell script. Optionally you can set a schedule to run them recurringly. Cronicle is nice and clean and does its job great.
Call Cronicle with REST on GitHub
#Cronicle also has a REST-API that offers basic tasks like start a job, check if it has finished, and get the log output. You can simply start a job from GitHub actions with the following script:
1name: Update dev environment
2
3on:
4 push:
5 branches: [ development]
6
7jobs:
8 call_api:
9 runs-on: ubuntu-latest
10
11 steps:
12 - name: HTTP Request Action
13 uses: fjogeleit/http-request-action@v1.5.0
14 with:
15 url: ${{ secrets.API_URL }}
16 method: 'POST'
17 data: >-
18 {
19 "id": "${{ secrets.API_ID }}" ,
20 "api_key": "${{ secrets.API_KEY }}"
21 }
22
Because it just starts the Job we don’t get any info on what happens. In the GitHub Actions log, you just see whether the API call worked and not the log we get from the task itself. There is no info on what goes on on the server.
This was a problem for another use case. I want to run a database install script in a local database when a Pull Request was created at GitHub. Ideally, the Pull-Request should then show if this Action-Run was successful or not and you should be able to jump to the log output to find out what is broken within GitHub.
The Plugin
#I concluded that the easiest solution would be to write a GitHub Actions-Plugin specifically for Cronicle with a simple API. Here is an example:
1name: run-tests-on-pr
2
3on:
4 pull_request:
5 types: [opened, synchronize, reopened]
6
7jobs:
8 install-db-objects:
9 runs-on: ubuntu-latest
10
11 steps:
12 - uses: phartenfeller/cronicle-job-action@v1.1.0
13 with:
14 cronicle_host: ${{ secrets.CRONICLE_HOST }}
15 event_id: ${{ secrets.CRONICLE_TEST_INSTALL_JOB_ID }}
16 api_key: ${{ secrets.CRONICLE_KEY }}
17 fail_on_regex_match: '[1-9]+ invalid object\(s\) remaining after last recompilation|ORA-[0-9]+|ERROR at line'
18 parameter_object: '{"branch": "${{ github.head_ref }}"}'
19
You can pass a regex that scans the output log and sets the run as failed for a match. If no regex is passed it just uses the Cronicle job status. You can also pass parameters that are used as env variables in Cronicle. In this case, I pass the branch of the pull request to check this branch out and run the script from there.
The run output gets automatically spooled to the GitHub action run history so you can easily check it there:
Feel free to try this plugin out if you need it and create issues in the repo if there are bugs / you miss a feature. You can find the GitHub repo here.